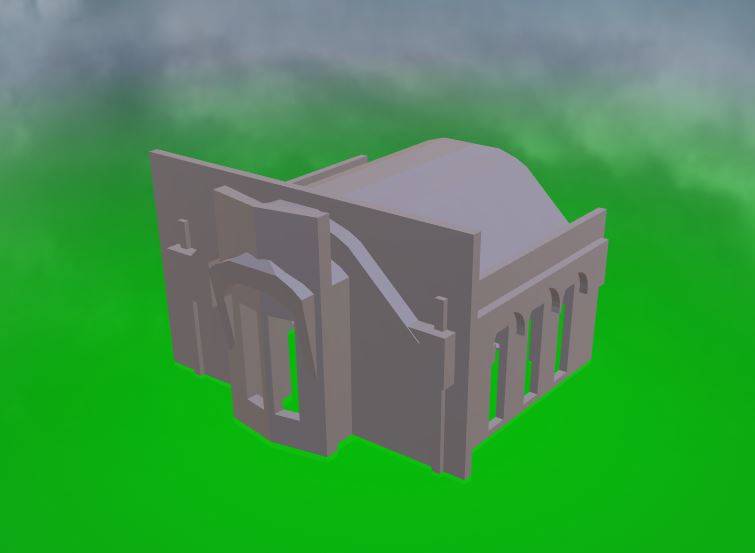
This is the 3rd tutorial in our Bablylon.JS series.
In the first two, we created a scene, added the WebVR capability, added a skybox, and finally the ground.
But what is an environment without music and sound effects?
To begin, here is our javascript from the first two tutorials:
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Tutorial 3 - Audio in Babylon js</title>
<!-- Babylon.js -->
<script src="https://preview.babylonjs.com/gltf_validator.js"></script>
<script src="https://preview.babylonjs.com/babylon.js"></script>
<script src="https://preview.babylonjs.com/loaders/babylonjs.loaders.js"></script>
<style>
html, body {overflow: hidden; width: 100%;
height: 100%; margin: 0; padding: 0; }
#renderCanvas { width: 100%; height: 100%;
touch-action: none; }
</style>
</head>
<body>
<canvas id="renderCanvas"></canvas>
<script>
var canvas = document.getElementById("renderCanvas");
var createScene = function() {
// Create scene
var scene = new BABYLON.Scene(engine);
// Default Environment
var environment = scene.createDefaultEnvironment({
createSkybox: false,
createGround: true,
groundSize: 1000,
groundColor: BABYLON.Color3.Green(),
enableGroundShadow: true,
groundYBias: 1
});
var skyMaterial = new BABYLON.StandardMaterial("skybox", scene);
skyMaterial.backFaceCulling = false;
skyMaterial.reflectionTexture = new BABYLON.CubeTexture("textures/skybox", scene);
skyMaterial.reflectionTexture.coordinatesMode = BABYLON.Texture.SKYBOX_MODE;
skyMaterial.diffuseColor = new BABYLON.Color3(0, 0, 0);
skyMaterial.specularColor = new BABYLON.Color3(0, 0, 0);
var skybox = BABYLON.MeshBuilder.CreateBox("skyBox", {size:1000.0}, scene);
skybox.material = skyMaterial;
// Enable VR
var vrHelper = scene.createDefaultVRExperience({createDeviceOrientationCamera:false});
vrHelper.enableTeleportation({floorMeshes: [environment.ground]});
var building = BABYLON.SceneLoader.Append("./", "vrchapel.glb", scene, function (meshes) {
scene.createDefaultCameraOrLight(true, true, true);
});
return scene;
};
var engine = new BABYLON.Engine(canvas, true, { preserveDrawingBuffer: true, stencil: true });
var scene = createScene();
engine.runRenderLoop(function () {
if (scene) {
scene.render();
}
});
// Resize
window.addEventListener("resize", function () {
engine.resize();
});
</script>
</body>
</html>
According to the documentation, the expected file type is mp3, but I found that wav file types also work without a problem.
Make sure that the audio file is located in the same or a sub-folder of the html file that will be loading it to play.
Now to load the audio file. I placed the instructions before the WebVR is loaded, immediately after the SkyBox is loaded:
// A little mood music
var music = new BABYLON.Sound("music", "mnkchant.mp3", scene, soundReady, {loop: true });
function soundReady() {
music.play();
}
Let’s break the instruction down. We begin by creating a variable to associate with the sound file. It is loaded into memory using the new BABYLON.Sound command. The parameters for the command are:
- The name of the sound (I used the same name as the variable for convenience)
- The URL/location of the sound file
- The scene to load the sound into (i.e. scene)
- Function to call when the sound is loaded and ready to play
- Options such as looping, volume, isPaused, etc
Once the sound is loaded and ready to play, the function soundReady is called which will play the music.
I found that this is great to get the music playing by default. When it is loaded in your server (local or a webserver), you will see an icon displayed in the top left corner:
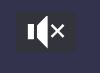
This is because modern browser automatically silence any audio that begins playing in the browser (and let’s be honest, this is a good thing).
Clicking on the audio will allow you to hear the audio that you loaded.
In a later tutorial we will add the controls for the audio portion of the environment.